Main Page

Document index

|
The visual light library creates a rendered image of multiple colored light sources with or without location handles for providing immediate feedback on lighting effects and a simple interface for adjustment.
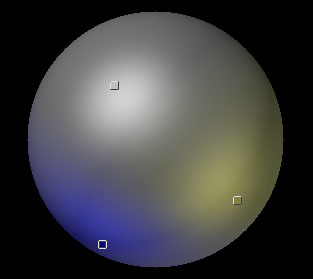
An example image generated by the visual light
The library is divided into three main function groups: initialization/setup, generation directives, and interaction.
- Initialization group
These functions setup the environment for visual light to operate in and provide results to the application.
int rlight_create(int pixelx, int pixely);
This function initializes the visual light library and allocates the image buffers for rendering.
void rlight_size(int pixelx, int pixely);
This function creates new image arrays and regenerates the image at the new size.
void rlight_destroy();
This function shuts down and releases all memory used by the library.
void rlight_surface(float diffuse, float spec, float gloss);
This function sets the surface properties for the sphere. This function does not cause a re-render of the image. Use rlight_image() for that.
void rlight_ambient(color *ambient);
This function sets the ambient light color for the scene.
By default this value is {0.1, 0.1, 0.1}.
void rlight_camera(svector *direction);
This function sets the camera direction. All light positions/directions are rotated through the camera. A full camera matrix is generated from the supplied direction and an up vector pointing up the Y axis. Don't count on any reasonable result if a camera direction pointing up or down the Y axis is supplied.
void rlight_marker(int display);
This function enables (display=1) or disables (display=0) visible light markers.
- Light directives
int rlight_add(svector *direction, color *lightcolor, float bright);
This function adds a new light to the lighting list. A rendering is called, and this new light is the selected light. This function returns the index of the new light, or zero if an error occured.
void rlight_image();
Rebuilts the rendering pages and final image. Used to intentionally re-render after changing ambient or surface properties.
- Interaction
int rlight_pick(int mousex, int mousey);
This function selects a nearest light in the image to the input point. These coordinates must be local to the image. The return code is the index of the selected light, zero if no light handle was close enough, and -1 if the point selected is not on the sphere. This function causes a re-render if the focus changes.
int rlight_set(int lightnumber);
This function sets the highlighted light to the index passed. The same index is returned if successful or zero if the index is invalid or an error occured. This function causes a re-render if the focus changes.
int rlight_move( |
int lightnumber, |
|
int mousex, int mousey, |
|
svector *result); |
This function projects the mouse coordinates into 3d and moves lightnumber. If the coordinates are off the sphere, the light is moved to the edge of the sphere closest to the coordinates. A normalized vector is saved in result of the new light direction. This function returns the light index if successful, or zero if an error occurred. The image is updated with the new light position.
int rlight_remove(int lightnumber);
This function removes the indicated light. This function returns the index number of the light removed or zero if the light index is invalid. All other lights are re-numbered to fill in any gaps. (ie light numbers can change often and are sequencial 1-n; calling rlight_remove(1) repeatedly will remove all the lights).
void rlight_change( |
int lightnumber, operation-code, |
|
svector *dir, |
|
color *c, float bright); |
This function provides access for changing the color and/or the direction of the light. The operation codes are:
0 - Change color & position
1 - Change position
2 - Change color/brightness.
- Globals
int rlvw_x, rlvw_y;
Indicates the current width and height of the image the library renders.
long *rlbit_final;
Points to the final image bitmap.
A sample minimal implementation would look something like:,
void drawlight(void)
{
svector v;
color c;
rlight_create(160,120);
rlight_surface(0.8, 0.5, 0.1);
v.x= 0.0, v.y= 5.0, v.z= 100.0;
rlight_camera(& v);
v.x= -2.0, v.y= 4.0, v.z= 1.0;
c.r= c.g= c.b= 1.0;
rlight_add(& v, & c, 0.75);
/** draw with rlbit_final ... */
rlight_destroy();
}
|